New Game - Sync Think
I have been working on a new game that is currently available for Alpha testers. The working title is ‘Sync Think’. The general idea is for players to submit a random word or phrase and then work together to converge on a single word. The faster you match with another player, the more points you’ll score. It is inspired by this improv game called Mind Meld. It is typically only played with two players, but my version supports 2-16 players. When more then 2 people are playing, two words from the myriad of guesses are chosen randomly to be the words for the next “level”. With many people playing, it is possible for some players to match and others not. Once you match, you’ll score points. It continues until everyone has matched or one player is left out. It will also stop after the maximum level has been reached (currently set at 7).
Example
Level | Player 1 Word | Player 2 Word |
---|---|---|
Random | Banana | Katana |
Level 1 | Sliced | Cut Fruit |
Level 2 | Snack | Snack |
In this example, the players matched after 2 levels.
Implementation Challenges
What defines a match? Is it an exact word match? Should typos count? Should things like swim
and swimming
count as matching? How do you handle when players get “close” but the words are completely different? How do you display all the guesses in a way that makes sense and shows the “journey”?
Exact word match
This is perhaps the most pure implementation of the “rules” but in practice I found this not to be much fun. You end up converging towards an idea and get close but it doesn’t “count” since they aren’t exactly the same word. Players end up a circling around the same idea, hoping to type the same word or eventually just “agreeing” to write the same thing so they can move on. To make the game less frustrating, I abandoned this strategy early on.
Should typos count?
I have another game called Canvas Clash which is a drawing game with 2 players drawing the same word and the others need to guess what is being drawn. The faster you guess, the more points you score. For this game, it was more fun if being close still counted to prevent frustration with typos. For that, I use the an algorithm called Damerau–Levenshtein distance to determine similarity/equality between the guess and actual word. I use a distance of 1 to allow for minor typos. Canvas Clash is more concerned with getting the general idea of what is being drawn rather than mandating perfect typing.
Here’s a few examples of what is ‘accepted’ as a typo
Target Word: Chair
Guess | Accepted | Reason |
---|---|---|
Chairs | ✅ | 's' was added at the end. Only 1 letter added to match. |
Char | ✅ | The letter 'i' is missing. Only 1 shift is required to match |
Chart | ❌ | The 't' is extra and the 'i' is missing. That's 2 substitutions. |
For Sync Think
, I don’t think this same approach would work as there are multiple words that are “close” but distinctly different things. Take for example the words
case
and chase
. These are only one substitution apart with the Damerau–Levenshtein
calculation but these are two quite distinct things. In Canvas Clash, this is more than likely a typo as you wouldn’t confuse a drawing of a case and chase so it can be accepted as an answer. If we can’t rely on simple typo checks, what else can we do?
Word Stemming
I googled around looking for a good way to normalize the strings in a way where they could be considered “equal”. I stumbled on this medium post related to String data normalization and similarity algorithms. It seemed to mostly be related to the normalizing the names of companies for exact matching or searching purposes. It describes using regular expressions for removing spaces, punctuation, and normalizing business extensions. I was able to take advantage of some of the techniques here to normalize the text but I was more interested in checking out the mentioned stemming algorithms.
What is it?
Stemming, also called suffix stripping, is a technique used to reduce text dimensionality. In short, that means distilling the word down to its meaningful ‘stem’ and throwing out the rest. Think of suffixes for making nouns plural or word endings that change the tense -ed
-ing
.
An example might be drive
-> driving
-> drove
. They are all more or less the same ‘stem’ but in different tenses. These should ‘match’ in the game. Word stemming also takes care of plurals so that would fix chair
vs chairs
etc.
Libraries
I first looked at the Lancaster algorithm but thought that it was a bit too aggressive (e.g. mice
and mic
would match but those are definitely two different things). I then looked at Porter and Snowball (Porter2). These also offer quite variety of languages which was a good match for Gametje. Luckily there is a nice java library from ~2002-2014 that provides some nice stemming techniques that are directly applicable in this game. The main downside of this library was that it had not been updated since ~2014 and was a manual download. The site leaves quite a lot to be desired and is definitely a product of its era. In my own projects, I am used to using maven for easy dependency management. I searched around to see if anyone had made it publicly available. Luckily I found someone who “modernized” it and published it on maven-central. Kudos to rholder for his repackaging of the library. That made it a simple declaration in my backend code and I was on my way. Using the library was quite simple. 7/9 languages that I support were also supported in the library. Only Polish and Slovakian were not supported.
Using an LLM to determine equality?
I currently use Open AI’s ChatGPT gpt-4o-mini
for my backend AI for generating responses in REQUIP
, SNAPTION
, and IMAGINARIAN
. I wondered if I could also use it with this new game to help determine if two words were actually the same exact thing. Here’s an example from my testing with some co-workers:
DEVELOPER
, SOFTWARE ENGINEER
, PROGRAMMER
.
These are all completely separate words, but more or less refer to the exact same thing. No amount of word-stemming or typo correction would mark these as a match. To a human, these are more or less the same thing. Without marking these as equal, it makes the next level a bit awkward as how do you find commonality between two things that are basically the same.
LLMs to the rescue!
Here’s a sample prompt I tried:
You are the judge in a game where players submit words or phrases. You have to determine if the words or phrases are either exactly the same or are referring to the same thing. An example would be 'Chair' and 'Stool'. These are two different words, but refer to something quite similar. When prompted with a number of words or phrases with each denoted in quotes, respond with the words that are the same. Leave out the words that are different. If none of them are the same, respond with INVALID.
It seemed to work fairly well but it adds more reliance on the AI for the game and potential cost if I need to ask it for “similar” matches very often. As my player base scales, this may introduce some extra cost. From a gameplay perspective, I may need to also consider reducing points if you get a similar match vs an exact/stemmed match. I also would need to explain the match in the UI that they were the “same” as deemed by the LLM. That also potentially brings up confusion if the LLM hallucinates or isn’t lenient enough. For now I have made it a flag on the backend and will experiment with the prompt a bit. I also need to worry about multiples of non-matching words if there is a large group of players.
e.g. TULIP
, GARDEN
FIELD
, FLOWER
There are 2 groupings there you might want it to match on but they all shouldn’t match each other.
Displaying guesses/matches
My original idea to display matches and guesses was to try to make a bunch of circles with the outer most ones showing the initial guesses and working your way inward to show when a match was found.
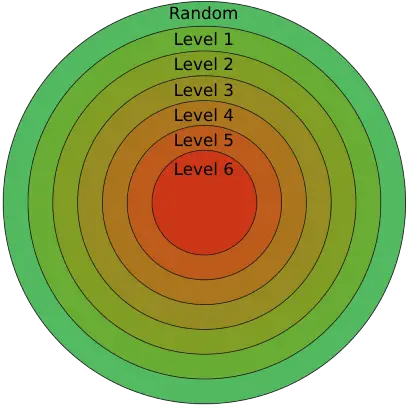
I still really like this idea, but it would be difficult to fit the actual guesses into each level and still have it look good, especially if you are playing with 5+ players. Keeping the words within the sliver of a circle, rotating them in just the right way with variable length and still having them fit. I decided against this for version 1 just to keep it simple.
Option 2 would be using a square grid:

This could work but doesn’t really make it easy to display all the guesses on their way to finding a match. Horizontal also doesn’t look that great on a mobile phone since most games are meant to be played in portrait. Doing it vertically could work it gave me an even better idea.
Starting far apart and converging together reminded me a tree-structure. The outer most guesses would be the leaves/branches, with the eventual match ending at the root of the tree. I found a similar javascript solution fairly quickly and thought it looked much better. It is easier to scale with the number of players and a “line of thinking” is easier to follow since the guesses are separated by space. Here’s an example:
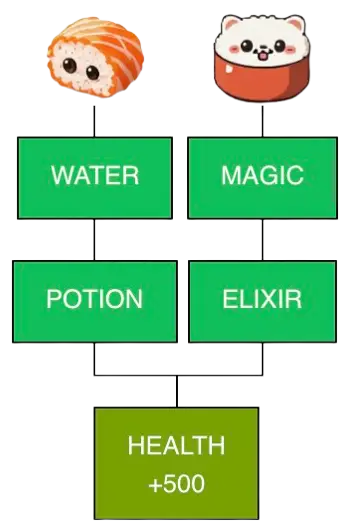
The main thing I struggle with is showing the context of each guess. With two players, it’ll show each level’s word and guesses but for 3+ players, your guess may not have been chosen as a “level word” so just following the line may not be totally accurate. I think I need to display each level’s chosen words on the side for full clarity but for now it will just show your guesses on the way to a match.
Getting the game out of Alpha
The game is currently still in alpha mode for a number of reasons. I have not finalized the gameplay loop and I am still trying to alleviate the pain when players get close but don’t find an exact match. I still need to go back and rephrase some of the text/instructions and do the translations for the various languages.
Looking forward to getting it out to everyone. Happy gaming!